Getting Started with C++ on Raspberry Pi
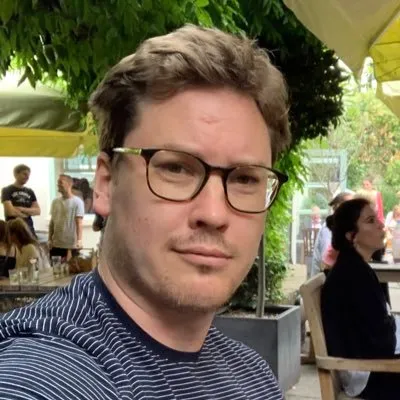
C++ is a powerful and versatile programming language widely used in system/software development, game programming, drivers, and more. The Raspberry Pi, with its flexible and accessible hardware, is an excellent platform for learning and experimenting with C++. This guide will walk you through the steps to set up and start programming with C++ on your Raspberry Pi.
Equipment Needed
- Raspberry Pi (any model, preferably Raspberry Pi 3 or 4)
- MicroSD card (at least 8GB)
- MicroSD card reader
- Internet connection
- Power supply for Raspberry Pi
Step 1: Prepare Your Raspberry Pi
Install Raspberry Pi OS
- Download Raspberry Pi OS:
Download the latest version of Raspberry Pi OS from the official Raspberry Pi website.
- Write the OS to the MicroSD Card:
Use Raspberry Pi Imager or Balena Etcher to write the Raspberry Pi OS image to your MicroSD card.
- Set Up the Raspberry Pi:
Insert the MicroSD card into your Raspberry Pi, connect it to a monitor, keyboard, and power it on. Follow the setup instructions to configure your Raspberry Pi.
- Update Your System:
Ensure your Raspberry Pi is up-to-date with the latest software and security patches.
sudo apt update
sudo apt upgrade
Step 2: Install the C++ Compiler
To compile and run C++ programs on your Raspberry Pi, you need to install the GNU Compiler Collection (GCC), which includes the g++ compiler for C++.
- Install g++:
sudo apt install g++
- Verify the Installation:
Check that g++ is installed correctly by checking its version.
g++ --version
You should see output displaying the version of g++ installed.
Step 3: Write Your First C++ Program
Create a C++ File
- Open a Text Editor:
You can use any text editor available on Raspberry Pi OS, such as Nano, Geany, or Vim. For this example, we will use Nano.
nano hello.cpp
- Write Your First C++ Program:
Enter the following code into the file:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
- Save and Exit:
Save the file and exit the text editor. In Nano, you can do this by pressing Ctrl+X
, then Y
to confirm, and Enter
to save.
Step 4: Compile and Run Your C++ Program
- Compile the Program:
Use g++ to compile the C++ program you just wrote.
g++ -o hello hello.cpp
This command tells g++ to compile hello.cpp
and output the executable named hello
.
- Run the Program:
Execute the compiled program.
./hello
You should see the output:
Hello, World!
Step 5: Explore More C++ Features
Once you have successfully run your first C++ program, you can start exploring more features and functionalities of the language. Here are a few ideas to get you started:
Variables and Data Types
#include <iostream>
int main() {
int myNumber = 5;
double myDouble = 5.99;
char myChar = 'D';
std::string myString = "Hello";
bool myBoolean = true;
std::cout << myNumber << std::endl;
std::cout << myDouble << std::endl;
std::cout << myChar << std::endl;
std::cout << myString << std::endl;
std::cout << std::boolalpha << myBoolean << std::endl;
return 0;
}
Control Structures
#include <iostream>
int main() {
int x = 10;
if (x > 0) {
std::cout << "x is positive" << std::endl;
} else {
std::cout << "x is not positive" << std::endl;
}
for (int i = 0; i < 5; ++i) {
std::cout << "i is " << i << std::endl;
}
return 0;
}
Functions
#include <iostream>
void printMessage() {
std::cout << "This is a function!" << std::endl;
}
int main() {
printMessage();
return 0;
}
Step 6: Use an Integrated Development Environment (IDE)
For more complex projects, you might want to use an Integrated Development Environment (IDE) that provides features like syntax highlighting, code completion, and debugging tools.
Install Geany
- Install Geany:
sudo apt install geany
- Launch Geany:
Open Geany from the application menu or by typing geany
in the terminal.
- Create and Manage Projects:
Use Geany to create and manage your C++ projects, write code, and compile programs.
Conclusion
By following these steps, you can set up and start programming with C++ on your Raspberry Pi. Whether you're learning C++ for the first time or using it for advanced projects, the Raspberry Pi provides a flexible and accessible platform for development. Experiment with more features, build projects, and enhance your programming skills with C++ on your Raspberry Pi.