Internet Speed Monitoring with Raspberry Pi - A Detailed Guide
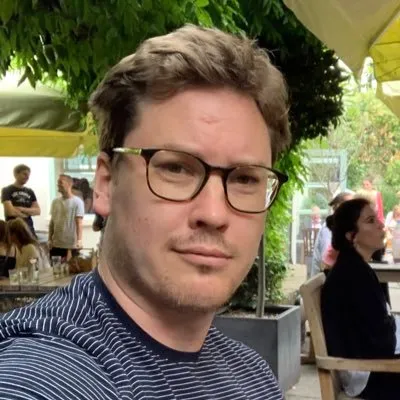
What is covered in this guide
-
Introduction: An introductory overview explaining the importance of monitoring internet speed and how a Raspberry Pi can be the ideal tool for this task. This section will also outline the scope and objectives of this blog post.
-
What is Raspberry Pi?: A thorough discussion on what Raspberry Pi is, its features, components, different models, and its various applications, specifically its capability to perform network monitoring tasks like internet speed tests.
-
Why Monitor Internet Speed?: An in-depth explanation of why monitoring internet speed is essential, covering use-cases in both personal and professional settings.
-
Setting Up Your Raspberry Pi:
- Hardware Requirements: A comprehensive list of all hardware needed to set up your Raspberry Pi for internet speed monitoring.
- Installing Raspberry Pi OS: Step-by-step guidance on installing Raspberry Pi OS, the official operating system for Raspberry Pi.
-
Installing Necessary Software:
- System Updates: How to update system packages for optimum performance.
- Speed Test Tools: Detailed instructions for installing speedtest-cli or other tools for performing internet speed tests.
-
Writing the Speed Test Script:
- Code Explanation: A line-by-line breakdown of the Python script that will perform the internet speed tests.
- Interpreting Results: Guidelines on how to understand and make use of the speed test data.
-
Optional: Storing Data Locally and Remotely:
- Local Storage: How to write speed test data to a local file such as CSV or JSON.
- Remote Storage: Methods to send this data to a remote server using APIs.
-
Automating the Speed Test:
- Cron Jobs: How to use cron jobs to automate your speed tests.
- Alternative Methods: Discussion of other scheduling options.
-
Data Visualization:
- Python Libraries: Using Python libraries like Matplotlib for graphical representation of your data.
- Web Dashboards: If applicable, how to set up a web-based dashboard for real-time data monitoring.
-
Optional: Sending Alerts:
- When and How: Criteria for sending alerts and example code snippets for Email and SMS notifications.
-
Troubleshooting and Common Issues:
- Network Issues: Solutions for common network-related problems.
- Software Issues: How to troubleshoot common software issues.
-
Conclusion: A summary of what this guide accomplishes, its practical applications, and how it empowers you to better understand your network.
-
Appendix:
- Glossary of Terms: Definitions of technical terms used throughout the guide.
- Additional Resources and Links: Recommend further reading materials, software tools, and communities for diving deeper into Raspberry Pi and network monitoring.
Introduction
Why Monitoring Internet Speed is Important
In our modern, hyper-connected world, a reliable and fast internet connection is more essential than ever. Whether you're working from home, streaming media, or managing a network of devices, knowing the speed and reliability of your internet can help you identify issues before they become problematic. Monitoring your internet speed can give you the data needed to negotiate with your Internet Service Provider (ISP), diagnose network issues, or decide on an upgrade. Essentially, what gets measured gets managed.
Raspberry Pi: The Perfect Tool for the Job
Enter Raspberry Pi, a low-cost and highly versatile computing platform that's perfect for this task. With its small form factor, energy efficiency, and powerful Linux-based operating system, a Raspberry Pi can easily run network monitoring tools 24/7 without breaking the bank. It's an accessible platform that invites both beginners and seasoned tech enthusiasts to experiment and build. Given its flexibility, you can even extend the project later to include more advanced features like data visualization or automated alerting systems.
Scope and Objectives
The aim of this blog post is to guide you through setting up your Raspberry Pi to automatically monitor your internet speed. We'll walk through the hardware and software requirements, go step-by-step in coding a Python script that will run the speed test, and set up automation so your Raspberry Pi performs the test at regular intervals. Optionally, we'll also explore storing this data and visualize it, so you can track your internet performance over time.
In summary, this guide seeks to offer a comprehensive yet easy-to-follow path to setting up internet speed monitoring using a Raspberry Pi. Whether you're a tech-savvy individual curious about your network performance or someone managing a more complex setup, this guide is designed to give you the tools and knowledge you need to keep your finger on the pulse of your internet connection.
Prerequisites
Before we dive into the actual process, let's take a moment to cover the essentials. Understanding the prerequisites will ensure you have everything you need to follow this tutorial smoothly.
Hardware Requirements
Raspberry Pi Model Recommendations
You can use almost any Raspberry Pi model for this project, but a Raspberry Pi 3 or newer is recommended for better performance and Wi-Fi support. The Raspberry Pi Zero W could also work if you're aiming for a minimal setup.
Other Required Components
- SD Card: Minimum 8GB, Class 10 recommended for better performance.
- Power Supply: 5V and at least 2A.
- Ethernet Cable: Optional but recommended for more accurate results.
- Wi-Fi or Ethernet Connection: To your network.
Software Requirements
Operating System
We'll be using Raspberry Pi OS (formerly known as Raspbian), which is the official OS provided by Raspberry Pi. You can download it from the Raspberry Pi website.
Libraries and Tools
- speedtest-cli: This is the command-line interface for testing internet bandwidth using speedtest.net.
-
Python Libraries: If you opt for storing data or sending alerts, Python libraries like
csv
,json
, orsmtplib
may be required. - Cron: For automation, we will be using the cron job scheduler.
Basic Knowledge Prerequisites
While this guide aims to be comprehensive and beginner-friendly, having some basic understanding of the following will be beneficial:
- Python: We'll be using Python for scripting. Basic knowledge of variables, loops, and functions will suffice.
- Linux: Familiarity with Linux command-line commands, as we'll be using the terminal to execute tasks.
- Networking: A basic understanding of how internet and local networking work can be helpful, but is not strictly necessary.
Setting up Your Raspberry Pi
Now that we've covered the prerequisites, let's get your Raspberry Pi up and running. This section will walk you through assembling the hardware, installing the Raspberry Pi OS, and performing the initial setup and configuration.
Assembling Hardware
What You'll Need
- Raspberry Pi (Model 3 or newer recommended)
- SD Card (preloaded with Raspberry Pi OS or empty)
- Power supply
- Ethernet Cable (optional)
- Monitor with HDMI input
- Keyboard and Mouse
- Insert the SD Card: Insert your SD card into the SD card slot on the Raspberry Pi.
- Connect Peripherals: Plug in your keyboard, mouse, and monitor using the appropriate ports on the Raspberry Pi.
- Network Connection: If you're using an Ethernet cable for a more stable connection, plug it into the Ethernet port. Otherwise, you'll connect to Wi-Fi later.
- Power Up: Finally, plug in the power supply to boot up the Raspberry Pi.
Installing Raspberry Pi OS
If your SD card is not preloaded with Raspberry Pi OS, you'll need to install it:
- Download Raspberry Pi OS: Download the latest version of Raspberry Pi OS from the official website.
- Flash SD Card: Use software like Balena Etcher to flash the downloaded OS image onto your SD card.
- Insert and Boot: Once the flashing process is complete, insert the SD card into your Raspberry Pi and power it on.
Initial Setup and Configuration
- Initial Boot: Upon first boot, you should see a setup wizard.
- Locale and Keyboard: Follow the wizard to set your locale, timezone, and keyboard settings.
- Wi-Fi Setup: If you're not using Ethernet, connect to your Wi-Fi network through the setup wizard.
- Update Software: It's always a good idea to start with updated software. Open a terminal and run the following commands:
sudo apt update
sudo apt upgrade
-
Enable SSH (Optional): If you plan to access your Raspberry Pi remotely, enable SSH via the Raspberry Pi Configuration menu (
sudo raspi-config
).
Installing Necessary Software
After setting up your Raspberry Pi, the next step is to install the software packages that will power your internet speed monitoring system. We'll focus on updating system packages, installing the speed test tool, and adding any Python libraries that may be needed for extended functionalities.
Updating System Packages
Before installing new software, it's good practice to update your system packages to their latest versions. Open a terminal on your Raspberry Pi and execute the following commands:
sudo apt update
sudo apt upgrade -y
This will fetch the list of available updates and then upgrade your installed packages.
Installing Speed Test Tools
There are several tools available for running speed tests, but we'll use speedtest-cli due to its simplicity and compatibility. To install it, run:
sudo apt install speedtest-cli
After the installation is complete, you can run a quick test to ensure it's working:
speedtest-cli
This command will perform a speed test and display the results in the terminal.
Optional: Installing Python Libraries
If you plan to extend the functionality of this project by storing data, sending alerts, or data visualization, certain Python libraries may be required. Here are some that could be useful:
CSV Library: For writing results to a CSV file.
pip install csv
JSON Library: For storing results in a JSON file.
pip install simplejson
SMTP Library: For sending email alerts.
pip install secure-smtplib
To install Python libraries, you may first need to install pip, the Python package installer:
sudo apt install python3-pip
Writing the Speed Test Script
We've reached the core part of this project—writing the Python script that will automate the internet speed tests. We'll go through the code step-by-step, helping you create a Python script that performs the test and stores the data.
Step-by-Step Code Explanation
Let's create a Python file named speed_test.py
to house our code. You can use any text editor or IDE that you're comfortable with.
Importing Libraries
First, we'll import the necessary libraries. For now, we'll stick with the basics.
import os
import subprocess
Running the Speed Test
We'll use Python's subprocess library to call the speedtest-cli tool and fetch the result.
def run_speed_test():
result = subprocess.run(['speedtest-cli', '--simple'], capture_output=True, text=True)
return result.stdout
This function will run the speed test and return the result as a string.
Complete Python Script
Here's the complete script combining all the steps:
import os
import subprocess
def run_speed_test():
result = subprocess.run(['speedtest-cli', '--simple'], capture_output=True, text=True)
return result.stdout
if __name__ == "__main__":
speed_test_result = run_speed_test()
print(speed_test_result)
Save this script, and run it using:
python3 speed_test.py
How to Interpret the Results
Once you run the script, you'll get output like:
Ping: 10 ms
Download: 50.34 Mbit/s
Upload: 12.34 Mbit/s
- Ping: This measures the latency or delay between your Raspberry Pi and the server. Lower values are better.
- Download: This measures the download speed—how quickly data can be pulled from the server to your Raspberry Pi. Higher values are better.
- Upload: This measures the upload speed—how quickly data can be sent from your Raspberry Pi to the server. Higher values are better.
You can use these metrics to gauge the quality of your internet connection at any given time.
Optional: Storing Data Locally and Remotely
Once your speed test script is up and running, you may want to store this data for long-term analysis or even send it to a remote server. In this section, we'll explore these advanced options.
Writing Data to a Local File
Storing your speed test results locally is straightforward. You can use either CSV or JSON format depending on your needs.
Storing in CSV
First, add import csv
at the top of your Python file. Then, modify your script to write to a CSV file.
import csv
def write_to_csv(data):
with open('speed_test_data.csv', 'a') as f:
writer = csv.writer(f)
writer.writerow([data['ping'], data['download'], data['upload']])
Storing in JSON
Alternatively, you can store the data in a JSON file. Add import json at the top of your script and add the following function:
import json
def write_to_json(data):
with open('speed_test_data.json', 'a') as f:
json.dump(data, f)
f.write('\n')
How to Structure the Data
Whichever method you choose, consider structuring the data with a timestamp for better analysis later. You could update the functions above to include timestamps.
Optional: Sending Data to a Remote Server
Sending your speed test results to a remote server opens up a world of possibilities for data analysis and alerting.
Using APIs
You can use Python libraries like requests to send POST requests to your API.
import requests
def send_to_server(data):
response = requests.post('http://your-server.com/api/speed-test', json=data)
Data Security Considerations
When sending data over the internet, always consider the following:
- Use HTTPS: Always use HTTPS to encrypt the data during transmission.
- Authentication: Implement some form of authentication to ensure that only authorized clients can send data.
- Data Integrity: Verify the integrity of received data on the server-side to protect against tampering.
This Markdown-formatted content should render correctly on platforms that support Markdown, such as GitHub or Markdown editors.
Automating the Speed Test
So far, we've written a Python script that runs a single speed test and explored options for storing the data. But wouldn't it be better if the Raspberry Pi could perform these tests automatically at specified intervals? Let's look at how to achieve this.
Using Cron Jobs to Automate the Tests
A cron job is a Linux command used for scheduling tasks to be executed at fixed times or intervals. It's perfect for our use case.
Setting up a Cron Job
Open the cron table for editing by running:
crontab -e
Add a new line at the end of the file to schedule your Python script. For example, to run the script every hour, add:
0 * * * * /usr/bin/python3 /path/to/your/speed_test.py
This will execute speed_test.py every hour on the hour.
Logging (Optional)
You may also want to log the output of the cron jobs for debugging purposes. Modify the cron line like this:
0 * * * * /usr/bin/python3 /path/to/your/speed_test.py >> /path/to/your/cron.log 2>&1
Alternative Scheduling Methods
While cron jobs are efficient and straightforward, there are other ways to schedule your tests if you need more flexibility or features.
Python schedule Library
The Python schedule library allows more complex scheduling right within your Python script. You can install it using pip:
pip install schedule
Then, within your Python script, you can do something like:
import schedule
def job():
# Your speed test code here
schedule.every(1).hours.do(job)
while True:
schedule.run_pending()
time.sleep(1)
Systemd Timers
Systemd timers offer another way to schedule regular tasks on your Raspberry Pi. They are generally more powerful and flexible but are also a bit more complex to set up.
Data Visualization
Collecting data is only the first part of the equation. To gain insights into your internet speed over time, you'll want to visualize this data. In this section, we will explore various methods for turning your raw data into informative graphs and dashboards.
Using Python Libraries like Matplotlib
Matplotlib is a widely-used Python library for data visualization. First, you'll need to install it:
pip install matplotlib
Example Code
Here's a simple Python code snippet to visualize speed test data stored in a CSV file.
import csv
import matplotlib.pyplot as plt
timestamps, download_speeds, upload_speeds = [], [], []
with open('speed_test_data.csv', 'r') as f:
reader = csv.reader(f)
for row in reader:
timestamps.append(row[0])
download_speeds.append(float(row[1]))
upload_speeds.append(float(row[2]))
plt.plot(timestamps, download_speeds, label='Download Speeds')
plt.plot(timestamps, upload_speeds, label='Upload Speeds')
plt.xlabel('Timestamp')
plt.ylabel('Speed (Mbit/s)')
plt.title('Internet Speed Over Time')
plt.legend()
plt.show()
This script reads from a CSV file that has timestamps, download speeds, and upload speeds, and then plots them using Matplotlib.
Web-based Dashboards
If you want real-time visualization accessible from anywhere, web-based dashboards are a great option.
Grafana
Grafana is a popular open-source platform for monitoring and observability. You can send your data to a database like InfluxDB and then use Grafana to create real-time dashboards.
Installation
Installing Grafana and InfluxDB is out of this blog post's scope, but plenty of guides are available online.
Data Transfer
You would send data from your Raspberry Pi to InfluxDB, usually via HTTP requests, and then configure Grafana to read this database.
Data Security
Whether you're visualizing locally or through a web-based dashboard, always remember to secure your data. Use HTTPS and authentication methods to protect sensitive information.
Optional: Sending Alerts
Automated speed tests and data visualization can provide a detailed picture of your internet performance, but what if you need immediate notification when something goes wrong? This section explores how to send alerts via Email and SMS when certain conditions are met.
When to Send Alerts
Before diving into the code, decide under what circumstances you want to send alerts. Here are some examples:
- Download speed drops below a certain threshold
- Upload speed is below a certain point
- High latency or ping time
Sending Email Alerts
Python's smtplib library allows us to send emails. First, add this import to your Python script:
import smtplib
Email Alert Function
Here's a sample function to send email alerts:
def send_email_alert(message):
server = smtplib.SMTP('smtp.gmail.com', 587)
server.starttls()
server.login('[email protected]', 'your-password')
server.sendmail('[email protected]', '[email protected]', message)
server.quit()
Security Note: Hardcoding your email and password in the script is not advisable. Consider using environment variables or a configuration file.
Implementing the Alert
You can add a condition in your speed test function to trigger this email alert.
if download_speed < 20: # Replace 20 with your desired threshold
send_email_alert(f'Low download speed detected: {download_speed} Mbit/s')
Sending SMS Alerts
To send SMS, you can use services like Twilio. First, you'll need to install the Twilio Python package:
pip install twilio
SMS Alert Function
Here's a sample function for sending SMS using Twilio:
from twilio.rest import Client
def send_sms_alert(message):
client = Client('Twilio_Account_SID', 'Twilio_Auth_Token')
client.messages.create(body=message, from_='+1234567890', to='+0987654321')
Implementing the Alert
Like the email alert, you can add a condition in your speed test function.
if upload_speed < 10: # Replace 10 with your desired threshold
send_sms_alert(f'Low upload speed detected: {upload_speed} Mbit/s')
Troubleshooting and Common Issues
While this project is designed to run as smoothly as possible, there are always some roadblocks you might encounter. In this section, we'll address some common issues related to the network, Raspberry Pi hardware, and software, along with ways to troubleshoot them.
Network Issues
Unreliable Speed Test Results
- Possible Cause: Network congestion or multiple devices using the network.
- Solution: Run tests during different times to rule out external factors.
Connectivity Loss
- Possible Cause: ISP outages or router issues.
- Solution: Check your router logs or contact your ISP for more details.
Raspberry Pi-specific Challenges
Inconsistent Readings
- Possible Cause: Resource limitations on the Raspberry Pi.
- Solution: Close unnecessary processes or consider upgrading your Raspberry Pi.
SD Card Corruption
- Possible Cause: Sudden power losses or improper shutdowns.
- Solution: Always shut down the Raspberry Pi properly, and consider using read-only filesystems.
Software Troubleshooting
Crontab not Running the Script
- Possible Cause: Incorrect cron syntax or wrong script path.
- Solution: Check the cron logs and verify your script's path.
Script Errors
- Possible Cause: Missing packages or syntax errors.
- Solution: Run the script manually to catch any errors in the terminal.
Data Not Saving
- Possible Cause: File permission issues.
- Solution: Make sure the script has permission to write to the directory where you're trying to save the data.
Conclusion
Congratulations, you've successfully built an automated system for monitoring your internet speed using a Raspberry Pi! Not only does this project give you real-time data about your internet performance, but it also provides options for alerts and data visualization.
Summary
- Automated Speed Testing: Scheduled, unattended speed tests with the Raspberry Pi.
- Data Storage: Options for storing data locally or on a remote server.
- Visualization and Analysis: Use Python libraries or web dashboards for insightful data visualization.
- Alerts: Immediate notifications via Email or SMS for subpar internet performance.
- Troubleshooting: Addressed common issues and their solutions to keep your system running smoothly.
Additional Resources
For those interested in taking this project to the next level or exploring other avenues, here are some resources that could be useful:
- Advanced Data Visualization with Grafana: In-depth guide on using Grafana for more complex visualizations.
- Using Prometheus for Monitoring: An alternative to Grafana for data monitoring.
- Raspberry Pi Documentation: Comprehensive guides and tutorials for different Raspberry Pi models.
- Twilio API Documentation: Explore more functionalities of Twilio for sending alerts.
By incorporating additional features or integrating with other systems, you can make this project as simple or as advanced as you'd like. Thank you for reading, and happy monitoring!
Appendix
This section serves as a repository for terms and additional resources that can help clarify concepts or offer more depth to the topics discussed in this blog post.
Glossary of Terms
- Cron Job: A time-based job scheduler in Unix-like operating systems for running commands at specific intervals.
- CSV (Comma-Separated Values): A file format used to store tabular data, such as a spreadsheet or database.
- ISP (Internet Service Provider): A company that provides internet access.
- Latency: A measure of time delay experienced in a system.
- Mbit/s (Megabits per second): A unit of data transfer speed.
- Ping: A network utility used to test the reachability of a host on an Internet Protocol (IP) network.
- SMTP (Simple Mail Transfer Protocol): A protocol for sending email messages between servers.
- Twilio: A cloud communications platform offering various services including SMS, voice, and video communication.
Additional Resources and Links
- Learn Python: An interactive tutorial for Python beginners.
- Linux Crontab Tutorial: A guide to understanding and setting up cron jobs in Linux.
- Matplotlib Documentation: Comprehensive guide and tutorials for Matplotlib.
- Raspberry Pi Official Site: Resources, forums, and products related to Raspberry Pi.
- Speedtest CLI Documentation: Official documentation for the Speedtest CLI tool.
Feel free to explore these resources for a deeper understanding and further exploration into network monitoring and Raspberry Pi projects.