How to Setup Serial Communication Over GPIO on Raspberry Pi
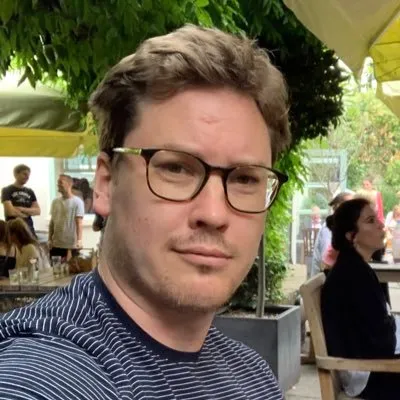
Serial communication is a fundamental aspect of many electronics projects, allowing devices to exchange data over a series of electrical pulses. The Raspberry Pi's GPIO (General Purpose Input/Output) pins include a UART (Universal Asynchronous Receiver/Transmitter) interface that can be used for serial communication. This guide will walk you through the steps to set up and use serial communication over GPIO on your Raspberry Pi.
Prerequisites
Before starting, ensure you have the following:
- Raspberry Pi (any model)
- MicroSD card with Raspberry Pi OS installed
- Stable internet connection
- Access to the command line (via monitor and keyboard or SSH)
- Jumper wires
- A serial device or another Raspberry Pi for testing
Step 1: Enable the Serial Interface
-
Open Raspberry Pi Configuration:
You can open the configuration tool from the desktop environment or via the terminal.
- On the desktop: Go to
Preferences
>Raspberry Pi Configuration
. - From the terminal:
- On the desktop: Go to
sudo raspi-config
-
Enable Serial Communication:
- Navigate to
Interface Options
. - Select
Serial
. - When prompted, choose
No
to the login shell over serial. - Choose
Yes
to enable the serial hardware.
- Navigate to
-
Reboot Your Raspberry Pi:
sudo reboot
Step 2: Identify the GPIO Pins for Serial Communication
The Raspberry Pi uses the following GPIO pins for UART serial communication:
- GPIO14 (TXD): Pin 8
- GPIO15 (RXD): Pin 10
- GND: Pin 6
Step 3: Connect Your Serial Device
Using jumper wires, connect your serial device to the Raspberry Pi's GPIO pins. For example, if you are connecting another Raspberry Pi:
- Raspberry Pi 1 (TXD) -> Raspberry Pi 2 (RXD)
- Raspberry Pi 1 (RXD) -> Raspberry Pi 2 (TXD)
- GND -> GND
Step 4: Install Minicom for Testing
Minicom is a text-based serial port communication program that you can use to test serial communication.
- Install Minicom:
sudo apt install minicom -y
- Open Minicom:
sudo minicom -b 9600 -o -D /dev/serial0
Replace 9600
with the baud rate of your serial device if it's different.
Step 5: Test Serial Communication
- Open Minicom on Both Devices:
If you are using two Raspberry Pi devices, open Minicom on both. Ensure the baud rate and device settings match.
- Send and Receive Data:
Type some text in the Minicom terminal on one Raspberry Pi. You should see the text appear on the Minicom terminal of the other Raspberry Pi, indicating successful serial communication.
Step 6: Write a Python Script for Serial Communication
To automate serial communication, you can use a Python script with the pyserial
library.
- Install PySerial:
pip install pyserial
- Create a Python Script:
Create a file named serial_test.py
and add the following code:
import serial
import time
ser = serial.Serial('/dev/serial0', 9600, timeout=1)
ser.flush()
while True:
if ser.in_waiting > 0:
line = ser.readline().decode('utf-8').rstrip()
print(line)
ser.write("Received: ".encode('utf-8') + line.encode('utf-8') + "\n".encode('utf-8'))
- Run the Python Script:
python serial_test.py
This script reads data from the serial interface and echoes it back with a "Received: " prefix.
Conclusion
By setting up serial communication over GPIO on your Raspberry Pi, you can interface with various serial devices for your electronics projects. Whether you're connecting sensors, microcontrollers, or other Raspberry Pi devices, serial communication provides a reliable way to exchange data. Enjoy experimenting with your new serial communication setup and explore the endless possibilities it offers for your projects!