Guide to Setting Up Digital Signage with Raspberry Pi
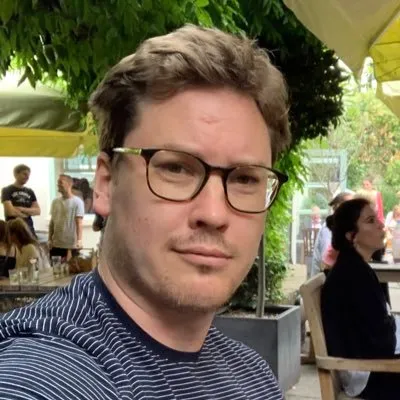
This guide aims to equip you with the knowledge to set up a digital signage system using a Raspberry Pi, tailored to all skill levels. We'll cover the steps from basic setup to advanced configurations, including code snippets and commands where relevant.
For Beginners: Basic Setup
- Installing Raspberry Pi OS
Download Raspberry Pi Imager: Visit the Raspberry Pi Downloads page and download the Raspberry Pi Imager for your operating system. Flash Raspberry Pi OS: Open the Imager, select the OS (choose Raspberry Pi OS Full for a complete set of features), select your SD card, and click "WRITE".
- Initial Raspberry Pi Setup
Insert the SD card into your Raspberry Pi, connect it to a monitor, keyboard, mouse, and power supply. Upon first boot, follow the setup wizard to configure your locale, network, and update your system.
sudo apt update
sudo apt full-upgrade
- Installing Digital Signage Software (Screenly OSE)
Open a terminal and enter the following commands to install Screenly OSE:
bash <(curl -sL https://www.screenly.io/install-ose.sh)
Follow the on-screen instructions to complete the installation.
For Intermediate Users: Enhancing Functionality
- Setting Up Remote SSH Access
Enable SSH through the Raspberry Pi configuration menu:
sudo raspi-config
Navigate to Interfacing Options > SSH and enable it.
Find your Raspberry Pi’s IP address:
hostname -I
From your computer, connect to your Raspberry Pi:
ssh pi@your_raspberry_pi_ip
- Automating Content Updates with Cron Jobs
Edit the crontab file to add your scheduled tasks:
crontab -e
Add a line to refresh your content. For example, to reboot daily at 2 AM:
0 2 * * * sudo reboot
For Advanced Users: Custom Solutions and Scaling
- Developing Interactive Displays
Python Script for GPIO Input:
import RPi.GPIO as GPIO
import os
GPIO.setmode(GPIO.BCM)
GPIO.setup(18, GPIO.IN, pull_up_down=GPIO.PUD_UP)
def display_content(channel):
os.system('DISPLAY=:0 chromium-browser http://yourcontent.com')
GPIO.add_event_detect(18, GPIO.FALLING, callback=display_content, bouncetime=300)
try:
input("Press Enter to exit\n")
except KeyboardInterrupt:
pass
finally:
GPIO.cleanup()
This script waits for a button press connected to GPIO 18 to open a web page in Chromium.
- Scaling with Network Management Tools
Ansible Playbook for Updating Software:
- hosts: digital_signage
become: yes
tasks:
- name: Update and upgrade all packages
apt:
update_cache: yes
upgrade: dist
This Ansible playbook can be used to update all packages on machines in the digital_signage group.
- Integrating Live Data Feeds
To display live data, you can use web technologies (e.g., JavaScript) to pull data from APIs and refresh content dynamically. Example:
<html>
<head>
<title>Live Data Digital Signage</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
function fetchData() {
$.getJSON('https://api.example.com/data', function(data) {
$('#content').html(data.content);
});
}
$(document).ready(function(){
fetchData();
setInterval(fetchData, 60000); // Refresh every 60 seconds
});
</script>
</head>
<body>
<div id="content">Loading...</div>
</body>
</html>
By following these steps and incorporating the provided code snippets, users of all levels can create and manage a dynamic digital signage system with Raspberry Pi. Whether you're setting up a simple display or developing a complex network of interactive signs, the flexibility of the Raspberry Pi and the power of its community offer endless possibilities.