How to Check GPIO Pin States on Raspberry Pi
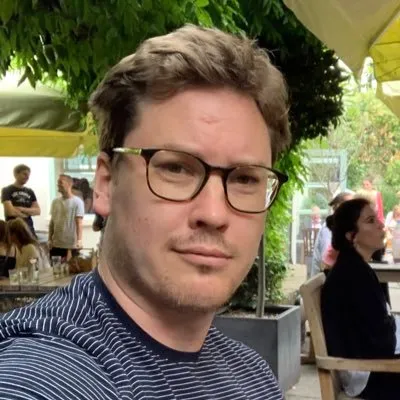
The General-Purpose Input/Output (GPIO) pins on a Raspberry Pi allow you to interface with a wide range of sensors, actuators, and other electronic components. Knowing how to check the state of these GPIO pins is crucial for troubleshooting and ensuring your projects run smoothly. This guide will show you how to check GPIO pin states on your Raspberry Pi using the command line and Python.
Equipment Needed
- Raspberry Pi with Raspberry Pi OS installed
- Internet connection
- Access to the command line
- GPIO-connected devices (e.g., LEDs, buttons)
Using the Command Line
Install WiringPi
WiringPi is a useful library that includes a command-line tool for interacting with GPIO pins.
- Install WiringPi:
Update your package list and install WiringPi:
sudo apt update
sudo apt install wiringpi
- Check GPIO Pin States:
Use the gpio readall
command to display the current state of all GPIO pins:
gpio readall
This command provides a table showing the pin numbers, modes, and current states (HIGH or LOW) of all GPIO pins.
Using sysfs Interface
The sysfs interface provides a method to interact with GPIO pins directly from the file system.
- Export the GPIO Pin:
Export the pin you want to check. Replace PIN_NUMBER
with the pin number you are interested in (BCM numbering).
echo PIN_NUMBER | sudo tee /sys/class/gpio/export
- Set the Pin Direction:
Set the pin direction to input:
echo "in" | sudo tee /sys/class/gpio/gpioPIN_NUMBER/direction
- Read the Pin State:
Read the state of the pin:
cat /sys/class/gpio/gpioPIN_NUMBER/value
The output will be 0
(LOW) or 1
(HIGH).
Using Python
Python provides a more flexible and programmable way to check GPIO pin states. The RPi.GPIO
library is commonly used for this purpose.
Install RPi.GPIO Library
- Install the RPi.GPIO library:
sudo apt install python3-rpi.gpio
Check GPIO Pin States with Python
- Create a Python Script:
Create a new Python script named check_gpio.py
:
nano check_gpio.py
- Add the Following Code:
import RPi.GPIO as GPIO
# Use BCM pin numbering
GPIO.setmode(GPIO.BCM)
# Define the GPIO pin number you want to check
pin_number = 17
# Set up the GPIO pin as an input
GPIO.setup(pin_number, GPIO.IN)
# Read the state of the pin
pin_state = GPIO.input(pin_number)
# Print the state of the pin
print(f"GPIO pin {pin_number} is {'HIGH' if pin_state else 'LOW'}")
# Clean up
GPIO.cleanup()
- Run the Script:
Save and close the file, then run the script:
python3 check_gpio.py
The script will print whether the specified GPIO pin is HIGH or LOW.
Conclusion
Checking the state of GPIO pins on your Raspberry Pi is an essential skill for debugging and interacting with your hardware projects. Whether you use command-line tools like WiringPi or the sysfs interface, or prefer the flexibility of Python, knowing how to read GPIO pin states ensures you can troubleshoot and verify your connections effectively. By mastering these techniques, you can confidently manage your Raspberry Pi projects and achieve reliable results.