How to Detect I2C Devices on Raspberry Pi
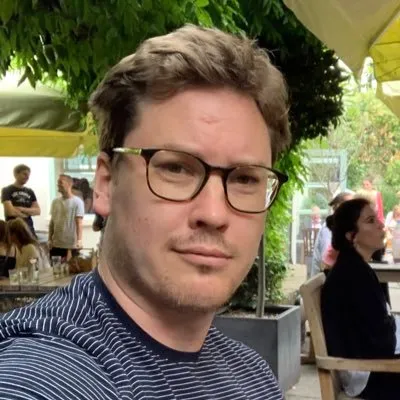
The Inter-Integrated Circuit (I2C) protocol is commonly used in electronics to communicate with sensors, displays, and other peripherals. Detecting I2C devices connected to your Raspberry Pi is a crucial step in many projects. This guide will show you how to enable the I2C interface on your Raspberry Pi and detect connected I2C devices.
Equipment Needed
- Raspberry Pi with Raspberry Pi OS installed
- I2C device (sensor, display, etc.)
- Internet connection
- Access to the command line
Enable I2C Interface
Before you can detect I2C devices, you need to enable the I2C interface on your Raspberry Pi.
- Open Raspberry Pi Configuration:
If you're using the Raspberry Pi desktop, go to the main menu and select Preferences
> Raspberry Pi Configuration
. If you're using the command line, use raspi-config
.
sudo raspi-config
- Navigate to Interface Options:
In the configuration tool, navigate to Interface Options
and select I2C
.
- Enable I2C:
Follow the prompts to enable the I2C interface.
- Reboot Your Raspberry Pi:
After enabling I2C, reboot your Raspberry Pi to apply the changes.
sudo reboot
Install I2C Tools
Next, install the I2C tools package, which includes utilities for detecting and interacting with I2C devices.
sudo apt update
sudo apt install -y i2c-tools
Connect Your I2C Device
Physically connect your I2C device to the GPIO pins on your Raspberry Pi. Typically, you'll connect the SDA (data) and SCL (clock) lines to the corresponding GPIO pins on the Raspberry Pi (GPIO2 and GPIO3 for SDA and SCL, respectively).
Detect I2C Devices
- List I2C Buses:
First, list the available I2C buses on your Raspberry Pi to verify the I2C interface is active.
ls /dev/i2c-*
You should see output like /dev/i2c-1
, indicating the I2C bus is available.
- Detect Connected Devices:
Use the i2cdetect
command to scan for I2C devices. Replace 1
with the appropriate bus number if different.
sudo i2cdetect -y 1
The output will be a grid showing the addresses of any detected I2C devices. For example:
0 1 2 3 4 5 6 7 8 9 a b c d e f
00: -- -- -- -- -- -- -- --
10: -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- --
20: -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- --
30: -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- --
40: -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- --
50: -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- --
60: -- -- -- -- -- -- -- -- 68 -- -- -- -- -- -- --
70: -- -- -- -- -- -- -- --
In this example, a device is detected at address 0x68
.
Interacting with I2C Devices
Once you've detected your I2C device, you can interact with it using various tools and libraries. For example, you can use the i2cget
and i2cset
commands to read from and write to I2C registers, or you can use Python libraries like smbus
for more complex interactions.
Example: Reading from an I2C Device
To read a byte from an I2C device at address 0x68
and register 0x00
:
sudo i2cget -y 1 0x68 0x00
Example: Using Python to Interact with I2C Devices
- Install smbus:
sudo apt install -y python3-smbus
- Python Script:
Create a Python script to read from your I2C device:
import smbus
import time
# Create an I2C bus object
bus = smbus.SMBus(1)
# I2C address of the device
address = 0x68
# Read a byte from register 0x00
data = bus.read_byte_data(address, 0x00)
print(f"Data: {data}")
Save the script and run it:
python3 script_name.py
Conclusion
Detecting I2C devices on your Raspberry Pi is a straightforward process once the I2C interface is enabled. With the help of i2c-tools
and Python libraries, you can easily interact with various sensors and modules, unlocking a wide range of possibilities for your projects. Whether you're building a weather station, a home automation system, or experimenting with new sensors, understanding how to detect and use I2C devices is an essential skill for any Raspberry Pi enthusiast.