How to Check Raspberry Pi Temperature from the Terminal
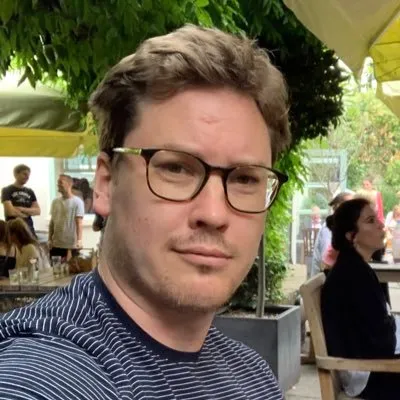
Monitoring the temperature of your Raspberry Pi is crucial to ensure it operates within safe limits and to prevent overheating, which can lead to throttling and damage. This guide will show you how to check the temperature of your Raspberry Pi directly from the terminal, helping you keep your device running smoothly.
Equipment Needed
- Raspberry Pi with Raspberry Pi OS installed
- Internet connection
- Access to the command line
Check Temperature Using Built-in Commands
Using vcgencmd
The Raspberry Pi comes with a built-in tool called vcgencmd
that allows you to query various system parameters, including temperature.
-
Open the Terminal:
Access the terminal on your Raspberry Pi. You can do this directly on the device or remotely via SSH.
-
Check the Temperature:
Run the following command to get the current temperature of your Raspberry Pi's CPU:
vcgencmd measure_temp`
The output will look something like this:
temp=45.2'C
Using /sys/class/thermal
You can also check the temperature by reading the value from the thermal zone files in the /sys
directory.
-
Open the Terminal:
Access the terminal on your Raspberry Pi.
-
Read the Temperature:
Run the following command to read the temperature:
cat /sys/class/thermal/thermal_zone0/temp
The output will be in millidegrees Celsius. For example, if the output is 45200
, it means the temperature is 45.2°C.
- Convert to Celsius:
If you prefer a more readable format, you can convert it to degrees Celsius:
echo $(($(cat /sys/class/thermal/thermal_zone0/temp) / 1000))'°C'
Using Python Script
For more advanced monitoring, you can use a Python script to periodically check and display the temperature.
- Create a Python Script:
Create a new Python script named check_temp.py
:
nano check_temp.py
- Add the Following Code:
import time
def get_cpu_temp():
with open("/sys/class/thermal/thermal_zone0/temp", "r") as f:
temp = f.read()
return float(temp) / 1000
while True:
temp = get_cpu_temp()
print(f"CPU Temperature: {temp:.2f}°C")
time.sleep(5)
-
Run the Script:
Save and close the file, then run the script:
python3 check_temp.py
This script will display the CPU temperature every 5 seconds.
Automating Temperature Monitoring
Setting Up a Cron Job
You can automate temperature monitoring and logging using a cron job.
- Create a Logging Script:
Create a script named log_temp.sh
:
nano log_temp.sh
- Add the Following Code:
#!/bin/bash
temp=$(vcgencmd measure_temp)
echo "$(date): $temp" >> /var/log/rpi_temp.log
- Make the Script Executable:
chmod +x log_temp.sh
- Set Up the Cron Job:
Open the cron table for editing:
crontab -e
Add the following line to log the temperature every hour:
0 * * * * /path/to/log_temp.sh
Save and exit the editor. The temperature will now be logged to /var/log/rpi_temp.log
every hour.
Conclusion
Monitoring the temperature of your Raspberry Pi is essential to maintain its performance and longevity. By using the built-in commands, a Python script, or setting up automated logging with cron jobs, you can ensure your Raspberry Pi operates within safe temperature ranges. Whether you are running a simple project or a complex server, keeping an eye on the temperature helps prevent potential overheating issues.