How to set up dht111 for Raspberry Pi
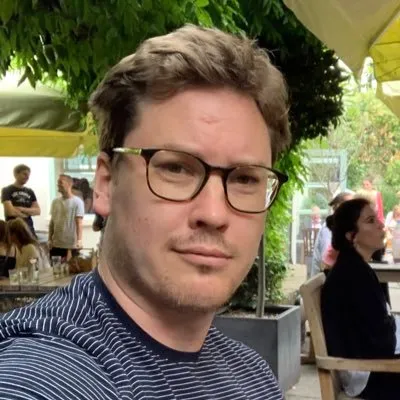
The DHT11 is a basic sensor that measures ambient temperature and relative humidity. It outputs a digital signal, making it relatively easy to interface with microcontrollers like the Raspberry Pi. While not the most precise sensor available (the DHT22 is its slightly more accurate sibling), the DHT11 is incredibly affordable and great for getting started.
What You'll Need
- Raspberry Pi: Any model with GPIO pins will work (Pi 2, 3, 4, Zero, etc.). Ensure it's set up with Raspberry Pi OS (or a similar Linux distribution) and connected to the internet.
- DHT11 Sensor: Often comes mounted on a small PCB with 3 pins. If you have a 4-pin version, you may need a 4.7kΩ to 10kΩ pull-up resistor.
- Jumper Wires: Female-to-female or female-to-male, depending on your setup.
- Breadboard (Optional): Recommended for easier and tidier connections.
Step 1: Wiring the DHT11 to Your Raspberry Pi
First, shut down your Raspberry Pi completely before connecting anything to the GPIO pins.
The DHT11 (3-pin module version) typically has pins labeled:
- VCC / + / 5V: Power supply input
- DATA / OUT / S: Data signal output
- GND / -: Ground
Use BCM pin numbering. Wiring example:
-
DHT11 VCC → Raspberry Pi 3.3V (Pin 1)
(Try 5V (Pin 2 or 4) if you have issues, but be cautious) - DHT11 DATA → Raspberry Pi GPIO 4 (Pin 7)
- DHT11 GND → Raspberry Pi Ground (Pin 6)
4-Pin Sensor Note
If you have a bare 4-pin sensor:
- Pin 1: VCC
- Pin 2: Data
- Pin 3: NC (Not Connected)
- Pin 4: GND
- Add a 4.7kΩ–10kΩ pull-up resistor between VCC and Data pin
Important: Double-check your wiring!
Step 2: Software Setup - Installing the Library
Boot your Raspberry Pi and open a Terminal window.
-
Update your package list:
sudo apt update
-
Install pip (if not installed):
sudo apt install python3-pip -y
-
Install the Adafruit DHT library:
sudo pip3 install Adafruit-CircuitPython-DHT
Note: For advanced projects, consider using a Python virtual environment.
Step 3: Writing the Python Script to Read the Sensor
Create a new Python file, e.g., dht11_reader.py
:
import time
import board
import adafruit_dht
# --- Configuration ---
SENSOR_TYPE = adafruit_dht.DHT11
SENSOR_PIN = board.D4
# --- End Configuration ---
try:
dhtDevice = SENSOR_TYPE(SENSOR_PIN)
print("DHT11 Sensor Initialized")
except Exception as error:
print(f"Error initializing sensor: {error.args[0]}")
print("Starting measurements (press CTRL+C to exit)...")
while True:
try:
temperature_c = dhtDevice.temperature
humidity = dhtDevice.humidity
if humidity is not None and temperature_c is not None:
temperature_f = temperature_c * (9 / 5) + 32
print(f"Temp: {temperature_c:.1f} C / {temperature_f:.1f} F | Humidity: {humidity:.1f}%")
else:
print("Sensor reading failed, trying again...")
except RuntimeError as error:
print(f"Runtime Error reading sensor: {error.args[0]}")
time.sleep(2.0)
continue
except Exception as error:
dhtDevice.exit()
raise error
time.sleep(2.0)
Explanation
- We import necessary libraries:
time
(for delays),board
(for GPIO pin definitions), andadafruit_dht
- We configure the
SENSOR_TYPE
(DHT11) andSENSOR_PIN
(GPIO 4 via board.D4) - We initialize the sensor using
adafruit_dht.DHT11(SENSOR_PIN)
- The
while True:
loop continuously reads the sensor -
dhtDevice.temperature
anddhtDevice.humidity
attempt to get the readings - We include checks (
if humidity is not None...
) because the DHT11 sometimes fails to provide a reading - We convert Celsius to Fahrenheit for convenience
-
try...except RuntimeError
is crucial for handling common read failures without crashing the script -
time.sleep(2.0)
is important; reading the DHT11 too frequently will cause errors
Step 4: Running the Script
Save the file and run it in the terminal:
python3 dht11_reader.py
You should see output like:
DHT11 Sensor Initialized Starting measurements (press CTRL+C to exit)... Temp: 23.0 C / 73.4 F | Humidity: 45.0% Temp: 23.0 C / 73.4 F | Humidity: 45.0% Sensor reading failed, trying again... Temp: 23.1 C / 73.6 F | Humidity: 44.8% Use CTRL+C to stop.
Troubleshooting Tips
- No Output / ImportError: Check if the library is installed and you're using python3
- RuntimeError: Timed out: Check wiring. Try 5V for VCC if 3.3V is unstable
-
Getting None or Fails Often:
- Check wiring
- Verify GPIO pin number
- Ensure proper power
- Try increasing sleep time to 2.5 or 3.0 seconds
-
Permission Errors:
- Try running with sudo
- Ensure user is in gpio group:
sudo adduser $USER gpio
Next Steps
Now that your Raspberry Pi can read temperature and humidity:
- Log data to a file
- Display data on an LCD
- Send data to services like ThingSpeak or Adafruit IO
- Set up alerts for threshold values
- Build a web server to show conditions
Happy tinkering!