Reading Serial Port Data with Raspberry Pi
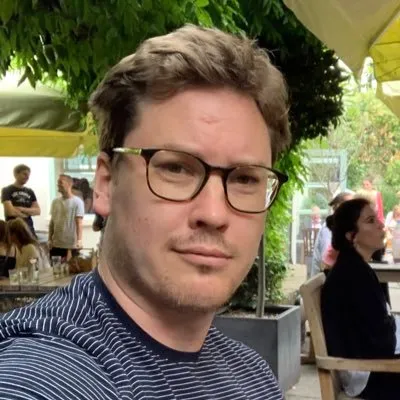
Serial communication is a critical component in many electronics projects, allowing devices to exchange information bit by bit over a wire. The Raspberry Pi includes UART (Universal Asynchronous Receiver/Transmitter) capabilities on its GPIO pins, making it capable of serial communication. This guide will explain how to read data from a serial port using a Raspberry Pi, enabling your projects to interact with various sensors, devices, or other microcontrollers.
Understanding Serial Ports on Raspberry Pi
The Raspberry Pi’s GPIO header provides access to UART serial communications, which are often used for communicating with other computers or peripherals. These capabilities can be harnessed for a multitude of applications, from telemetry systems to home automation networks.
Preparing Your Raspberry Pi
Before you start reading data from a serial port, make sure your Raspberry Pi is correctly set up:
-
Enable Serial Interface: You need to enable the UART interface on your Raspberry Pi. This can be done via the
raspi-config
tool:
sudo raspi-config
Navigate to Interfacing Options > Serial Port and enable it. Answer 'No' to the login shell over serial and 'Yes' to the serial hardware interface.
- Update and Upgrade Your Raspberry Pi: Ensure that your Raspberry Pi is running the latest software:
sudo apt update sudo apt full-upgrade -y
Equipment and Connections
- Serial Device: This could be another microcontroller, a GPS module, or any serial-enabled device.
- Wiring: Connect the TX pin of the serial device to the RX pin on the Raspberry Pi, and vice versa. Don’t forget to connect the GND (ground) pins between the Raspberry Pi and the serial device.
- Voltage Levels: Ensure that the voltage levels between the Raspberry Pi and the serial device are compatible. Raspberry Pi GPIO operates at 3.3V.
Reading Serial Data
Step 1: Install Python and PySerial
Python is an excellent choice for handling serial data due to its simplicity and powerful libraries. Install Python and the PySerial package, which provides easy handling of serial communication:
sudo apt install python3 python3-pip sudo pip3 install pyserial
Step 2: Write a Python Script to Read Serial Data
Create a new Python script that reads data from the serial port:
import serial import time # Setup serial connection ser = serial.Serial('/dev/serial0', 9600, timeout=1) # Open serial port at 9600 baudrate try: while True: if ser.in_waiting > 0: line = ser.readline().decode('utf-8').rstrip() # Read a line and decode it from bytes to string print(line) # Print the line time.sleep(0.1) except KeyboardInterrupt:print("Program terminated!") ser.close() # Close serial port
This script continuously reads lines of text from the serial port and prints them to the console. Adjust the serial port settings (/dev/serial0
and 9600
) as necessary for your configuration.
Conclusion
Reading data from a serial port with a Raspberry Pi opens a wide array of possibilities for integrating various hardware into your projects. Whether you are building a data logger, a communication interface, or just experimenting, understanding how to manage serial communication effectively is a valuable skill. This guide has set you up to start incorporating serial data into your Raspberry Pi projects, expanding both your knowledge base and your project's capabilities.