Advanced Computer Vision Concepts with Raspberry Pi and OpenCV [Series]
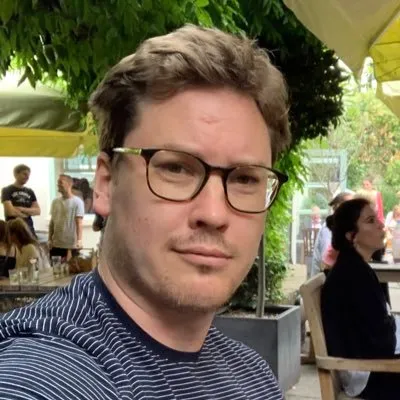
Series Quick Links
- OpenCV Basics & Introduction
- OpenCV Image Processing Fundamentals
- OpenCV Object Detection and Tracking
- OpenCV Advanced Computer Vision
- OpenCV Practical Applications of Computer Vision
- OpenCV Performance Optimization in Computer Vision
- OpenCV Future Trends and Application in Computer Vision
Introduction
Welcome to the third installment in our series on Raspberry Pi and OpenCV! After covering the basics of image processing and diving into object detection and tracking, we're now ready to explore some advanced computer vision concepts. In this article, we'll focus on facial recognition, gesture recognition, and optical character recognition (OCR). Let's dive right in!
Part 10: Facial Recognition
Implementing a Basic Facial Recognition System
Facial recognition has become ubiquitous in various applications, from security systems to personalized user experiences. OpenCV provides robust tools to implement facial recognition easily. It's not just about identifying a face; it's about enhancing user interaction and security measures.
Haar Cascades for Face Detection
The first step in facial recognition is detecting faces within an image or video feed. OpenCV's Haar Cascades are often used for this purpose. Haar Cascades work by training the algorithm to recognize specific features in faces, such as the eyes, nose, and mouth, making it a reliable method for face detection.
Here's a simple example:
import cv2
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
cap = cv2.VideoCapture(0)
while True:
_, img = cap.read()
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
faces = face_cascade.detectMultiScale(gray, 1.1, 4)
for (x, y, w, h) in faces:
cv2.rectangle(img, (x, y), (x+w, y+h), (255, 0, 0), 2)
cv2.imshow('Face Detection', img)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
Eigenfaces for Face Recognition
After detecting faces, the next step is to recognize them. One common method is using Eigenfaces. OpenCV provides the face.EigenFaceRecognizer_create()
function to implement this. Eigenfaces work by reducing the dimensionality of face images, making it easier to compare and recognize faces.
model = cv2.face.EigenFaceRecognizer_create()
model.train(np.asarray(training_data), np.asarray(labels))
_, result = model.predict(face)
Part 11: Gesture Recognition
Detecting and Interpreting Hand and Body Gestures
Gesture recognition is another fascinating area of computer vision that has applications in gaming, healthcare, and human-computer interaction. It involves detecting and interpreting hand or body movements, which can be particularly useful for touchless interfaces or sign language interpretation.
Skin Color Segmentation
One simple approach to hand gesture recognition is skin color segmentation. You can convert the image to HSV color space and then filter out skin-colored regions. This method is effective but can be sensitive to lighting conditions.
hsv = cv2.cvtColor(frame, cv2.COLOR_BGR2HSV)
mask = cv2.inRange(hsv, lower_skin, upper_skin)
Contour Analysis
After obtaining the skin mask, you can find the contour of the hand and use it to recognize different gestures. Contour analysis allows you to identify the shape and orientation of the hand, which can be mapped to specific commands or actions.
contours, _ = cv2.findContours(mask, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
cnt = max(contours, key=lambda x: cv2.contourArea(x))
cv2.drawContours(frame, [cnt], 0, (0, 255, 0), 3)
Part 12: Optical Character Recognition (OCR)
Reading Text from Images or Video Feeds
Optical Character Recognition (OCR) is the technology used to convert different types of documents, such as scanned paper documents, PDF files, or images captured by a digital camera, into editable and searchable data. OpenCV can be used in conjunction with OCR libraries like Tesseract to read text from images or video feeds. This is incredibly useful for applications like automated data entry, license plate recognition, or translating text from signs in real-time.
Here's a simple example using Python's pytesseract library:
import pytesseract
image = cv2.imread('text_image.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
text = pytesseract.image_to_string(gray)
print(text)
In this example, we read an image containing text, convert it to grayscale, and then use Tesseract's image_to_string
function to recognize the text.
Conclusion
In this article, we've explored some advanced topics in computer vision, including facial recognition, gesture recognition, and optical character recognition. These advanced techniques open up a world of possibilities for creating intelligent and interactive applications. Whether you're building a security system that recognizes authorized users, a virtual game that responds to hand gestures, or a smart scanner that can read and interpret text from documents, the tools and techniques you've learned here will serve as a solid foundation for your projects. Happy coding!