Image Processing Fundamentals with Raspberry Pi and OpenCV [Series]
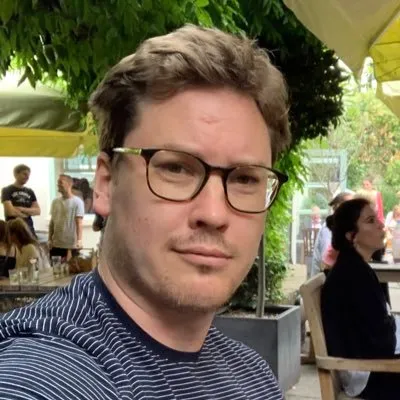
Series Quick Links
- OpenCV Basics & Introduction
- OpenCV Image Processing Fundamentals
- OpenCV Object Detection and Tracking
- OpenCV Advanced Computer Vision
- OpenCV Practical Applications of Computer Vision
- OpenCV Performance Optimization in Computer Vision
- OpenCV Future Trends and Application in Computer Vision
Introduction
Welcome to the second article in our series on Raspberry Pi and OpenCV! Having covered the basics of setting up your Raspberry Pi and writing your first OpenCV program, it's time to delve deeper into the world of image processing. In this comprehensive guide, we'll explore basic image operations, color spaces and transformations, as well as image filtering techniques. So, buckle up and get ready for an exciting journey into the realm of computer vision!
Part 4: Basic Image Operations
Reading Images
Reading an image file into your OpenCV program is the first step in any image processing task. The imread
function in OpenCV makes this incredibly simple. Here's a quick example:
import cv2
image = cv2.imread('example.jpg')
cv2.imshow('Example Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
Code Explanation:
In this example, the imread
function reads an image file named example.jpg
and stores it in the variable image
. The imshow
function then displays this image in a window.
Writing Images
Saving an image in OpenCV is just as straightforward as reading one. The imwrite
function allows you to save an image to disk. Here's how you can do it:
cv2.imwrite('example_copy.jpg', image)
Code Explanation:
The above line will save the image stored in the variable image
as example_copy.jpg
.
Manipulating Images
Once you've read an image into your program, you can perform various operations to manipulate it. These operations can range from simple tasks like cropping and resizing to more complex tasks like rotation and translation.
Cropping
Cropping an image involves selecting a rectangular region of an image and removing everything outside this region. In OpenCV, you can crop an image using simple array slicing.
cropped_image = image[50:200, 150:300]
Resizing
Resizing is often necessary when you're working with images of different dimensions. The resize
function in OpenCV allows you to change the dimensions of an image easily.
resized_image = cv2.resize(image, (300, 200))
Part 5: Color Spaces and Transformations
Understanding RGB
RGB stands for Red, Green, and Blue, the three primary colors of light. In an RGB image, each pixel's color is represented as a combination of these three colors. OpenCV stores RGB images as multi-dimensional NumPy arrays, but note that OpenCV reads images in BGR format, not RGB.
Splitting and Merging Channels
You can split an RGB image into its individual color channels using the split
function and merge them back using the merge
function.
blue, green, red = cv2.split(image)
merged_image = cv2.merge([blue, green, red])
Exploring HSV
HSV stands for Hue, Saturation, and Value. This color space is often used in image processing applications because it separates the chromatic information (hue) from the luminance information (value).
Converting Between RGB and HSV
You can convert an RGB image to HSV using the cvtColor
function.
hsv_image = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
Working with Grayscale
Grayscale images are simpler than color images because they contain only intensity information. Converting an image to grayscale can simplify the image processing pipeline.
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
Part 6: Image Filtering Techniques
Gaussian Filter
Gaussian filtering is used for blurring images and removing noise. It uses a Gaussian function to create a filter matrix which is then applied to the image.
gaussian_blur = cv2.GaussianBlur(image, (15, 15), 0)
Sobel Filter
The Sobel filter is used for edge detection. It operates by computing the gradient magnitude of an image at each pixel.
sobel_x = cv2.Sobel(gray_image, cv2.CV_64F, 1, 0, ksize=3)
sobel_y = cv2.Sobel(gray_image, cv2.CV_64F, 0, 1, ksize=3)
Canny Edge Detection
The Canny edge detector is another algorithm for edge detection but is more complex than the Sobel filter. It works by detecting areas of the image where there is a rapid change in intensity.
edges = cv2.Canny(gray_image, 100, 200)
Conclusion
In this article, we've covered a lot of ground in the world of image processing with Raspberry Pi and OpenCV. We've learned how to read, write, and manipulate images, explored different color spaces, and looked at various image filtering techniques. These are the building blocks that will enable you to develop more complex and exciting computer vision applications. So go ahead, experiment with what you've learned, and take your projects to the next level!