Raspberry Pi and OpenCV - A Comprehensive Guide for Beginners [Series]
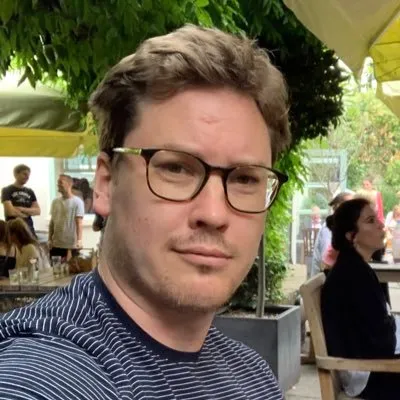
Series Quick Links
- OpenCV Basics & Introduction
- OpenCV Image Processing Fundamentals
- OpenCV Object Detection and Tracking
- OpenCV Advanced Computer Vision
- OpenCV Practical Applications of Computer Vision
- OpenCV Performance Optimization in Computer Vision
- OpenCV Future Trends and Application in Computer Vision
Introduction
Welcome to this all-in-one guide that aims to give you a comprehensive introduction to the fascinating world of Raspberry Pi and OpenCV. If you're a beginner looking to dive into the realm of computer vision, you've come to the right place. This guide will cover the basics of what Raspberry Pi and OpenCV are, how to set them up, and how to write your first OpenCV program on Raspberry Pi. So let's get started!
Part 1: The Basics
What is Raspberry Pi?
Raspberry Pi is a small, affordable, single-board computer developed by the Raspberry Pi Foundation. Initially designed to promote computer science education, these tiny machines have found their way into various types of projects, from simple DIY tasks to complex industrial applications.
Key Features of Raspberry Pi
- Affordable: One of the most significant advantages of using Raspberry Pi is its cost-effectiveness. With prices starting as low as $35, it's accessible for students, hobbyists, and developers alike.
- Versatile: The Raspberry Pi comes with a set of GPIO (General Purpose Input/Output) pins, allowing it to interface with a wide range of external hardware components, from LEDs and buttons to sensors and motors.
- Strong Community Support: One of the best things about Raspberry Pi is the community that surrounds it. There are countless forums, blogs, and tutorials available, making it easier for newcomers to find help and resources.
What is OpenCV?
OpenCV, which stands for Open Source Computer Vision Library, is an open-source computer vision and machine learning software library. Created by Intel in 1999, OpenCV has become the go-to library for all things related to computer vision. Whether you're building a facial recognition system or a self-driving car, chances are you'll use OpenCV.
Key Features of OpenCV
- Comprehensive Library: OpenCV offers a wide range of both classic and state-of-the-art computer vision algorithms. From basic image processing techniques to complex machine learning models, OpenCV has it all.
- Cross-Platform: One of the strengths of OpenCV is its cross-platform capabilities. Whether you're working on a Windows PC, a Mac, or a Raspberry Pi, OpenCV has got you covered.
- Multiple Language Support: OpenCV provides bindings for different programming languages, including Python, Java, and C/C++. This makes it accessible to a broad audience, from beginners to experts.
Why Use Them Together?
The combination of Raspberry Pi and OpenCV offers a powerful yet cost-effective solution for developing computer vision applications. Here's why they make a great pair:
- Cost-Effectiveness: Raspberry Pi's affordability and OpenCV's open-source nature make it possible to develop sophisticated computer vision applications without breaking the bank.
- Versatility: The hardware interfacing capabilities of Raspberry Pi, combined with the extensive algorithms available in OpenCV, offer endless possibilities for real-world applications.
- Accessibility: Both Raspberry Pi and OpenCV have extensive documentation and community support. This makes it easier for even beginners to dive into complex computer vision projects.
Part 2: Setting Up Your Raspberry Pi for OpenCV
Before diving into the coding part, it's essential to set up your Raspberry Pi correctly. Here's what you'll need:
Hardware Requirements
- Raspberry Pi board: Any model should work, but newer versions offer better performance.
- MicroSD card: At least 8GB is recommended.
- Power supply: A standard micro USB power supply should suffice.
- Optional: Camera module: If you're planning to work on image capture or video streaming, you'll need a camera module compatible with Raspberry Pi.
Software Installation
Setting up the software involves a few steps:
- Install Raspbian: Download and install the latest version of Raspbian, the official OS for Raspberry Pi.
-
Update Packages: Before installing new software, it's a good idea to update the existing packages. You can do this by running
sudo apt update && sudo apt upgrade
in the terminal. -
Install OpenCV: Installing OpenCV can be as simple as running
sudo apt install python3-opencv
in the terminal. This will install a pre-compiled package, which is the quickest way to get started.
Part 3: Your First OpenCV Program on Raspberry Pi
Introduction
Now that you've set up your Raspberry Pi and installed OpenCV, it's time to dive into the exciting part—writing code! In this section, we'll walk you through creating a simple Python script that uses OpenCV to capture video from a camera connected to your Raspberry Pi. We'll explain each line of code, so you'll not only know how to do it but also understand how it works.
Writing the Code
Setting Up Your Development Environment
Before we start writing code, make sure you have a text editor or Integrated Development Environment (IDE) installed on your Raspberry Pi. You can use any text editor, but IDEs like PyCharm or Visual Studio Code offer more features like code completion, debugging, and syntax highlighting.
Creating a New Python File
Open your text editor or IDE and create a new Python file. You can name it anything, but for this example, let's call it capture_video.py
.
Understanding the Code Structure
Here's the code snippet you'll be using:
import cv2
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
cv2.imshow('Frame', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
Code Explanation
-
import cv2
: This line imports the OpenCV library, which we installed earlier. -
cap = cv2.VideoCapture(0)
: Initializes the camera. The argument 0 indicates the default camera. If you have multiple cameras, you can select them by changing this number. -
while True:
: This is an infinite loop that will keep running until we explicitly break it. It's responsible for capturing the video frames. -
ret, frame = cap.read()
: Captures a single frame from the camera.ret
is a boolean that indicates if the frame was successfully captured, and frame contains the image data. -
cv2.imshow('Frame', frame)
: Displays the capturedframe
in a window named 'Frame'. -
if cv2.waitKey(1) & 0xFF == ord('q'):
: Waits for the user to press the 'q' key. Once pressed, the loop will break. -
cap.release() and cv2.destroyAllWindows()
: These lines release the camera and close any OpenCV windows.
Running the Program
Saving the Code
Once you've pasted the code into your text editor or IDE, save the file as capture_video.py
.
Executing the Script
Open the terminal, navigate to the folder where you saved capture_video.py, and run the following command:
python3 capture_video.py
What to Expect
A new window should appear, displaying the live video feed from your camera. This window will continue to display the video until you decide to close it. To do so, simply press the 'q' key, and the application will terminate, releasing all resources.
Troubleshooting and Tips
If you encounter any issues while running the program, here are some common troubleshooting tips:
- Camera not detected: Make sure your camera is correctly connected to the Raspberry Pi and is compatible with it.
- Syntax errors: Double-check your code to ensure there are no typos or syntax errors.
- High CPU usage: Video capture can be CPU-intensive. If you experience performance issues, consider optimizing your code or using a Raspberry Pi model with better performance capabilities.
Conclusion
Congratulations! You've just written and run your first OpenCV program on a Raspberry Pi. While this is a simple example, it lays the foundation for more complex and exciting computer vision projects. From here, you can expand into areas like object detection, facial recognition, or even machine learning. The sky's the limit, and you're now equipped with the basic knowledge to start exploring it. Happy coding!
Final Thoughts
Congratulations, you've just taken your first steps into the world of Raspberry Pi and OpenCV! This guide aimed to give you a solid start, but remember, this is just the tip of the iceberg. Both Raspberry Pi and OpenCV offer endless possibilities, limited only by your imagination. So go ahead, experiment, build, and most importantly, have fun learning!
Feel free to leave any questions or comments below, and stay tuned for more exciting tutorials on Raspberry Pi and OpenCV!