Performance Optimization in Computer Vision with Raspberry Pi and OpenCV [Series]
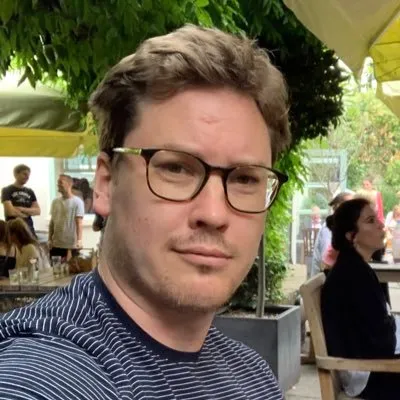
Series Quick Links
- OpenCV Basics & Introduction
- OpenCV Image Processing Fundamentals
- OpenCV Object Detection and Tracking
- OpenCV Advanced Computer Vision
- OpenCV Practical Applications of Computer Vision
- OpenCV Performance Optimization in Computer Vision
- OpenCV Future Trends and Application in Computer Vision
Introduction
Welcome to another installment in our series on Raspberry Pi and OpenCV! As we delve deeper into the world of computer vision, performance optimization becomes increasingly important. Whether you're building a real-time object tracking system or a complex facial recognition application, performance is key to ensuring that your system runs smoothly and efficiently.
In this article, we'll focus on various techniques to optimize the performance of your computer vision projects. We'll cover how to increase frame rates, efficiently store and retrieve images, and choose the most efficient algorithms for your tasks. Let's get started!
Part 13: Increasing Frame Rates
Techniques like Frame Throttling and Multi-threading
Frame Throttling
Frame throttling is a technique where you limit the number of frames processed per second (FPS) to improve the overall performance and reduce CPU usage. This is particularly useful in applications where real-time processing is not critical.
Here's a simple example using Python's time
library to throttle the frame rate to 30 FPS:
import cv2
import time
cap = cv2.VideoCapture(0)
desired_fps = 30
while True:
start_time = time.time()
ret, frame = cap.read()
Your processing code here
cv2.imshow('Frame', frame)
end_time = time.time()
elapsed_time = end_time - start_time
sleep_time = max(1./desired_fps - elapsed_time, 0)
time.sleep(sleep_time)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
Multi-threading
Multi-threading is another technique to improve performance by parallelizing tasks. Python's threading
library can be used to run multiple threads concurrently.
Here's a simple example where we use a separate thread for capturing frames:
import cv2
import threading
cap = cv2.VideoCapture(0)
def capture_frames():
while True:
ret, frame = cap.read()
Your processing code here
capture_thread = threading.Thread(target=capture_frames)
capture_thread.start()
Your main code here
capture_thread.join()
cap.release()
cv2.destroyAllWindows()
Part 17: Efficient Image Storage and Retrieval
Using Data Compression and Caching
Data Compression
Storing raw images can consume a lot of disk space, especially when dealing with high-resolution images or video feeds. Data compression techniques like JPEG or PNG compression can be used to reduce the storage requirements.
Here's how you can save an image using JPEG compression with OpenCV:
cv2.imwrite('image.jpg', frame, [cv2.IMWRITE_JPEG_QUALITY, 90])
Caching
Caching is another technique to improve performance by storing frequently used data in memory. This is particularly useful when you're dealing with a set of images that are used repeatedly.
Here's a simple example using Python's lru_cache
to cache images:
from functools import lru_cache
@lru_cache(maxsize=32)
def read_image(path):
return cv2.imread(path)
image1 = read_image('image1.jpg')
image2 = read_image('image2.jpg')
Part 18: Algorithmic Optimizations
Choosing Efficient Algorithms and Implementations
The choice of algorithm can significantly impact the performance of your application. For example, using a Haar Cascade for face detection is computationally less expensive than using a Deep Neural Network, although the latter may provide better accuracy.
Algorithm Selection
When selecting an algorithm, consider the following:
- Computational Complexity: Choose algorithms with lower time complexity for real-time applications.
- Hardware Compatibility: Some algorithms are optimized for specific hardware, like GPUs.
- Accuracy vs Speed: Sometimes a less accurate but faster algorithm might be more suitable for your application. Efficient Implementations
Many algorithms have multiple implementations, and some are more optimized than others. Libraries like OpenCV often provide multiple methods for the same task, and it's essential to choose the most efficient one for your specific needs.
For example, OpenCV provides different methods for resizing images, and some are faster than others:
resized = cv2.resize(image, (width, height), interpolation=cv2.INTER_LINEAR)
resized = cv2.resize(image, (width, height), interpolation=cv2.INTER_CUBIC)
Conclusion
Performance optimization is crucial for building efficient and robust computer vision applications. In this article, we've explored various techniques to improve the performance of your projects, from increasing frame rates through throttling and multi-threading to efficient image storage and algorithm selection. Implementing these techniques will not only make your applications faster but also more scalable and reliable. Happy coding!